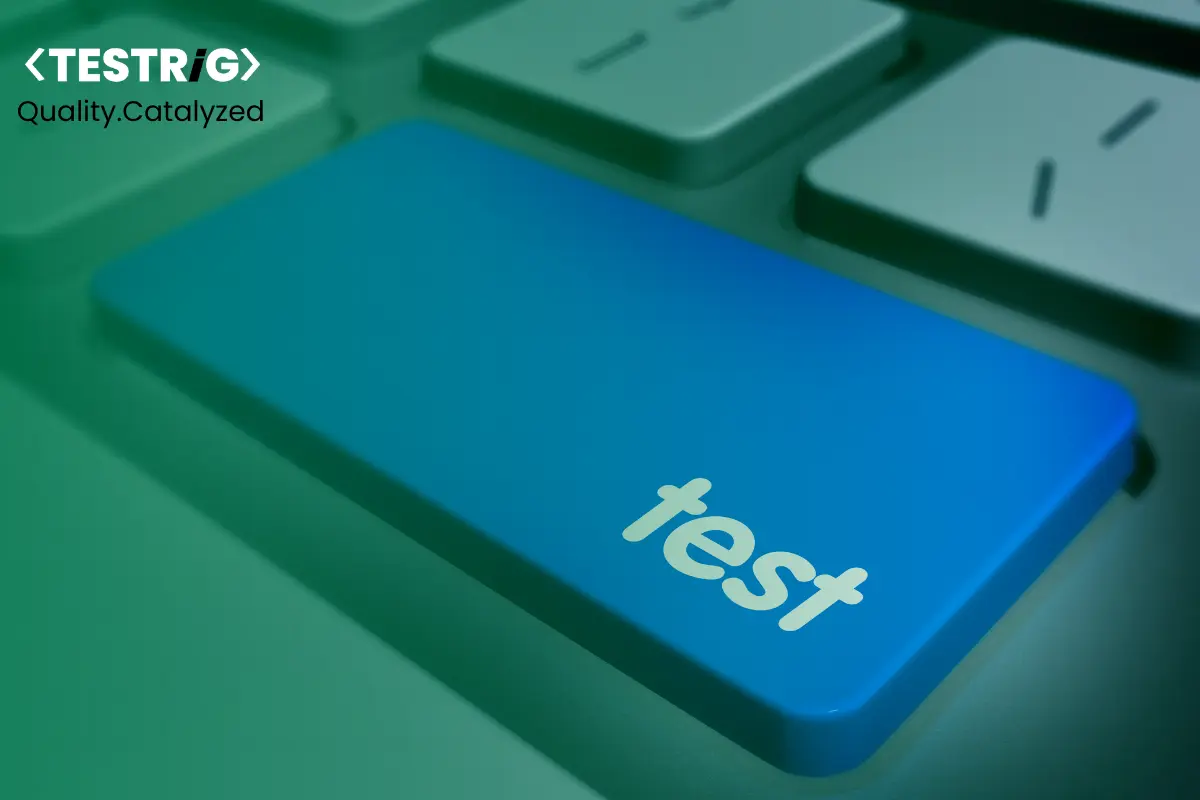
If you’re writing end-to-end (E2E) tests, Cypress is a top choice, but are you using it to its full potential? The secret to efficient, maintainable, and robust test automation lies in mastering Cypress commands—both built-in and custom.
Unlike Selenium, Cypress runs directly in the browser, providing real-time feedback, automatic waiting, and a rich API that makes test writing intuitive. However, without leveraging the right commands, your tests can become slow, flaky, or hard to maintain.
Cypress is the preferred tool for QA engineers performing end-to-end testing on modern web applications.Cypress offers a much deeper API that can help you build advanced, robust, and scalable test automation frameworks.
Core Cypress Commands
1. cy.get()
Fetches DOM elements using CSS selectors. It’s the cornerstone of DOM interaction.
cy.get(‘.submit-button’).click();
Tip: Chain .should() or .as() for assertions and aliasing.
2. cy.contains()
Finds an element with specific text, regardless of its position in the DOM.
cy.contains(‘Submit’).click();
Use Case: Ideal for buttons or links where selectors are dynamic.
3. cy.click()
Simulates a click on the selected element.
cy.get(‘#login-button’).click();
Tip: Use .click({ force: true }) if the element is hidden.
4. cy.type()
Types into an input or textarea field.
cy.get(‘#username’).type(‘qa@testrig.com‘);
Pro Tip: Add { delay: 100 } for simulating slower typing.
5. cy.select()
Selects an option from a dropdown.
cy.get(‘select’).select(‘India’);
Use Case: Works with <select> tags and handles options by label or value.
6. cy.scrollTo()
Scrolls to a specific part of the page.
cy.get(‘#footer’).scrollTo(‘bottom’);
Use Case: Useful for lazy-loaded content or verifying sticky elements.
7. cy.reload()
Reloads the current page.
cy.reload();
Use Case: Test app behavior on full reloads.
8. cy.url()
Gets the current URL.
cy.url().should(‘include’, ‘/dashboard’);
Use Case: Validates route changes and redirections.
9. cy.title()
Gets the page title.
cy.title().should(‘eq’, ‘Dashboard – MyApp’);
Use Case: Checks correct document titles after navigation.
10. cy.visit()
Navigates to a specified URL.
cy.visit(‘/login’);
Pro Tip: Use with baseUrl in Cypress config for cleaner code.
Advanced State Management & Optimization
11. cy.session()
Optimizes login by caching user sessions.
cy.session(‘login’, () => {
cy.visit(‘/login’);
cy.get(‘#email’).type(‘qa@testrig.com’);
cy.get(‘#password’).type(‘123456’);
cy.get(‘form’).submit();
});
Benefit: Significantly reduces test execution time.
12. cy.clearCookies() / cy.clearLocalStorage()
Cleans up between tests to avoid state leakage.
cy.clearCookies();
cy.clearLocalStorage();
Tip: Place in beforeEach() or afterEach() hooks.
13. cy.window() / cy.document()
Access browser-native objects.
cy.window().then((win) => {
expect(win.localStorage.getItem(‘token’)).to.exist;
});
Use Case: Verify session or token-based data.
API & Network Testing
14. cy.intercept()
Intercepts HTTP requests and mocks responses.
cy.intercept(‘GET’, ‘/api/users’, { fixture: ‘users.json’ }).as(‘getUsers’);
Tip: Use with cy.wait(‘@getUsers’) to validate response times and content.
15. cy.wait()
Waits for a specific amount of time or an alias.
cy.wait(2000); // Not recommended
cy.wait(‘@getUsers’); // Recommended
Best Practice: Avoid static waits. Use cy.intercept() + cy.wait(‘@alias’) for reliable sync.
16. cy.request()
Sends HTTP requests outside the browser.
cy.request(‘POST’, ‘/api/login’, { email: ‘qa@testrig.com’, password: ‘123456’ });
Use Case: API testing or setting test preconditions.
Cross-Origin and External Interactions
17. cy.origin()
Allows interaction with domains other than the app under test.
cy.origin(‘https://auth.example.com’, () => {
cy.get(‘input#username’).type(‘user’);
cy.get(‘input#password’).type(‘pass’);
cy.get(‘form’).submit();
});
Use Case: Login workflows involving SSO or OAuth.
18. cy.task()
Executes Node.js scripts for backend processes.
cy.task(‘resetDatabase’);
Use Case: Database seeding, email verification, or reading files.
File Operations & Debugging
19. cy.readFile() / cy.writeFile()
Handles reading and writing of files.
cy.readFile(‘cypress/fixtures/data.json’);
Use Case: Use for configuration or exporting test results.
20. cy.screenshot()
Captures screenshots during tests.
cy.screenshot({ capture: ‘viewport’ });
Tip: Automatically run on failure for visual debugging.
21. cy.exec()
Executes shell commands in the terminal.
cy.exec(‘npm run db:seed’);
Use Case: Useful in CI pipelines for setup or teardown.
Custom Commands & Functional Programming
22. Cypress.Commands.add()
Defines a reusable custom command.
Cypress.Commands.add(‘login’, () => {
cy.visit(‘/login’);
cy.get(‘#email’).type(‘qa@testrig.com’);
cy.get(‘#password’).type(‘123456’);
cy.get(‘form’).submit();
});
Benefit: DRY principle for repetitive tasks.
23. cy.wrap()
Wraps objects for use in Cypress chains.
const data = { email: ‘qa@testrig.com’ };
cy.wrap(data).its(’email’).should(‘contain’, ‘@’);
Use Case: Enables assertion chaining on non-DOM objects.
24. cy.then()
Allows callbacks in test chains.
cy.get(‘.price’).then(($price) => {
const amount = parseFloat($price.text().replace(‘$’, ”));
expect(amount).to.be.lessThan(100);
});
Tip: Use for complex calculations or conditional logic.
24. cy.invoke()
Calls a function on a selected element.
cy.get(‘input’).invoke(‘val’).should(‘eq’, ‘value’);
Use Case: Useful for manipulating jQuery elements.
25. cy.its()
Accesses nested object properties.
cy.wrap({ user: { name: ‘Priti’ } }).its(‘user.name’).should(‘eq’, ‘Priti’);
Use Case: Makes object-based assertions simpler.
End Note;
Mastering these advanced Cypress commands empowers you to go far beyond basic UI testing. Whether you’re streamlining test execution with cy.session(), validating backend processes using cy.task(), or building scalable custom workflows with Cypress.Commands.add(), each command enhances your ability to create fast, stable, and maintainable test suites.
Incorporating these techniques into your testing strategy will not only reduce flakiness and boost confidence in deployments but also foster a more developer-friendly QA environment. Keep exploring Cypress documentation and continuously iterate on your test framework to stay ahead in the test automation curve.
Need Help With Cypress Test Automation?
Testrig Technologies offers robust Cypress automation testing services for modern applications. From framework setup to CI/CD integration, we ensure your QA process is fast, reliable, and scalable.