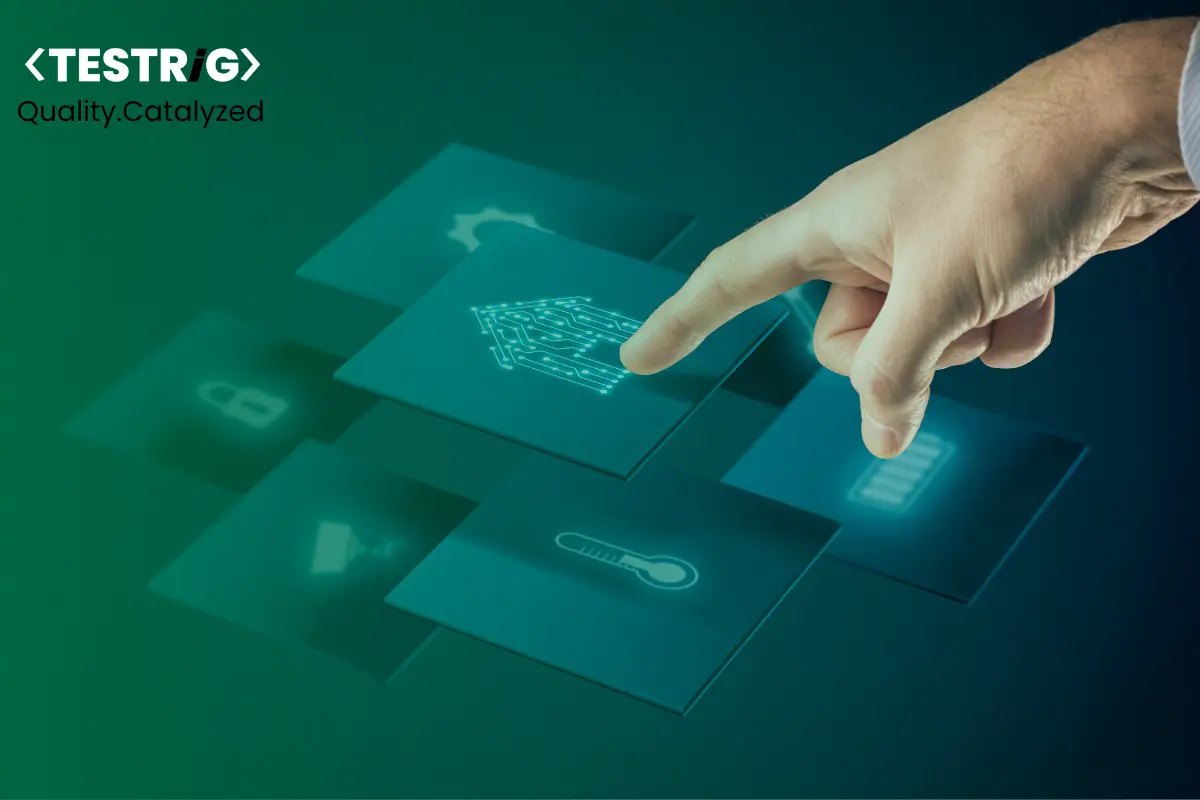
Welcome back to our Playwright series! In our last blog, we explored How Playwright Enhances Cross-Browser Testing Efficiency, highlighting how this powerful automation tool ensures seamless compatibility across different browsers. Now, we dive deeper into Playwright commands -line capabilities.
With Playwright’s powerful command-line interface (CLI), you can orchestrate complex test scenarios, debug with precision, and automate interactions across different browsers—all with just a few commands. But are you truly unlocking its full potential?
This guide goes beyond the basics, equipping you with the most essential Playwright commands that will supercharge your testing efficiency, minimize flakiness, and ensure rock-solid execution.
Top Most Essential Playwright Commands
Running Tests Efficiently
1. Run All Tests : To execute all test cases within your Playwright test suite, use:
npx playwright test
This command runs all available test files in your project and generates a detailed test report.
2. Run a Specific Test File: To execute a single test file, specify the filename:
npx playwright test filename.spec.ts
Replace filename.spec.ts with the actual test file name to focus execution on a particular test case.
3. Run Multiple Test Files: You can execute multiple specific test files simultaneously by listing them:
npx playwright test file1.spec.ts file2.spec.ts
This is particularly useful for targeted regression testing.
4. Run Tests Matching a Specific Pattern: To run test files that follow a specific naming convention or pattern:
npx playwright test filename*
This command executes all test files that match the provided pattern, making it convenient to categorize and run grouped tests.
5. Running Tests with Specific Tags : Playwright allows you to filter and execute tests based on tags using the –grep option. This is useful for categorizing tests like smoke, regression, or slow tests.
-> Run Tests with a Specific Tag: To execute only tests containing @smoke in their title, use:
npx playwright test –grep @smoke
-> Exclude Tests with a Specific Tag : To skip tests tagged as @slow, run:
npx playwright test –grep-invert @slow
-> Tagging Tests in Playwright : You can categorize tests using tags within test() descriptions:
test(‘User login should work @smoke’, async ({ page }) => {
await page.goto(‘https://example.com’);
await expect(page).toHaveTitle(‘Example’);
});
5. Running Tests in Debug Mode: Debugging is crucial for resolving flaky tests. Playwright provides an interactive debug mode:
npx playwright test –debug
This command launches the Playwright inspector, allowing you to step through test executions, inspect elements, and analyze failures interactively.
-> Slow Down Test Execution for Better Visibility : If you need to observe UI interactions more clearly, use the –slow-mo option to slow down execution:
npx playwright test –slow-mo=1000
This slows down each action by 1000 milliseconds (1 second), making it easier to debug UI behaviors.
Combining both options provides maximum control over debugging, ensuring smoother issue identification and resolution.
6. Disabling Parallel Execution : Playwright allows you to control test parallelism without adding extra lines of code. You can adjust the number of workers directly in the command or configure it in playwright.config.ts.
-> Run Tests Sequentially (Single Worker Mode) : To execute tests one at a time, use:
npx playwright test –workers=1
This is useful for debugging or when tests have dependencies that require sequential execution.
-> Run Tests with a Custom Number of Workers: To specify the number of parallel workers, simply adjust the worker count:
npx playwright test –workers=2
This allows controlled parallel execution, balancing speed and stability.
Set Workers in Playwright Config
You can also define the number of workers in playwright.config.ts:
export default defineConfig({
workers: 2, // Adjust as needed
});
-> Run Tests with Playwright’s UI Mode : For an interactive test execution experience, use:
npx playwright test –ui
This launches Playwright’s UI mode, where you can visually inspect test execution, debug failures, and re-run specific tests interactively.
7. Running Tests in Headless or Headed Mode : By default, Playwright runs in headless mode. To launch a visible browser, use:
npx playwright test –headed
To explicitly run in headless mode, use:
npx playwright test –headless
This flexibility is useful when debugging UI interactions.
8. Running Tests in Different Browsers : Playwright supports multiple browsers. To execute tests in a specific browser, use:
npx playwright test –project=chromium
npx playwright test –project=firebox
This ensures comprehensive cross-browser testing.
9. Recording and Replaying Tests : To generate test scripts using code recording, use:
npx playwright codegen example.com
This opens a browser and records user interactions, generating Playwright code automatically.
10. Capturing Traces for Better Debugging : Tracing is a powerful Playwright feature that helps analyze test failures by capturing the entire execution flow.
-> Enable Full Tracing: To record detailed traces for all test runs, use:
npx playwright test –trace on
This captures step-by-step execution, including network requests, screenshots, and console logs.
-> Capture Traces Only on Test Failures : To save traces only when a test fails, preventing unnecessary storage usage, use:
npx playwright test –trace=retain-on-failure
This ensures efficient debugging while keeping test logs manageable.
->View Saved Traces : After a test run, you can inspect the recorded traces using:
npx playwright show-trace trace.zip
This launches Playwright’s Trace Viewer, allowing you to replay and analyze test execution.
By leveraging tracing effectively, you can identify issues faster, improve debugging efficiency, and optimize your test automation workflow.
Validations and Assertions
11. Using Assertions for Test Validations: Playwright offers built-in assertion capabilities to validate UI elements and behaviors:
await expect(page).toHaveText(‘Expected Text’);
await expect(page.locator(‘#elementID’)).toBeVisible();
await expect(page).toHaveAttribute(‘href’, ‘https://example.com‘);
These assertions help ensure that web pages render correctly, and elements are functioning as expected.
Handling UI Interactions
12. Handling Alerts and Popups : To interact with browser alerts, prompts, or confirmation dialogs, use:
page.on(‘dialog’, async dialog => {
console.log(dialog.message());
await dialog.accept(); // or dialog.dismiss();
});
This ensures smooth test execution by handling unexpected popups.
13. Waiting for Elements to Load : Handling dynamic elements requires appropriate wait conditions to prevent flaky tests:
page.waitForSelector(‘#elementID’,{state: ‘visible’};
await page.waitForTimeout(3000); // Waits for 3 seconds
await page.waitForLoadState(‘networkidle’);
Using waitForSelector or waitForLoadState ensures elements are ready before interacting with them, improving test stability.
14. Capturing Screenshots for Debugging : Screenshots help in debugging test failures and capturing evidence:
await page.screenshot({ path: ‘screenshot.png’ });
Additionally, to capture a full-page screenshot, use:
await page.screenshot({ path: ‘fullpage.png’, fullPage: true });
Screenshots can also be captured per test using Playwright’s trace feature:
npx playwright test –trace=on
This allows for detailed debugging by reviewing screenshots within Playwright’s Trace Viewer, enhancing test reporting and analysis.
15. Emulating Mobile Devices
Playwright allows simulating mobile devices for responsive testing using built-in device presets:
const context = await browser.newContext({ …devices[‘iPhone 12’] });
This enables accurate testing of web applications on different screen sizes, viewports, and user agents without manually defining configurations. Playwright provides a wide range of predefined device presets, ensuring comprehensive mobile compatibility testing.
Final Thoughts
Mastering Playwright isn’t just about knowing the commands—it’s about optimizing your testing strategy. The right execution flow can speed up test cycles, improve reliability, and catch bugs before they reach production.
By integrating these essential Playwright commands into your workflow, you can fine-tune your test automation, ensure flawless execution, and boost efficiency. Whether you’re debugging flaky tests, handling alerts, or ensuring cross-browser compatibility, Playwright has the power to make testing seamless.
So, go ahead—experiment, refine, and push the limits of test automation with Playwright!